Hi pandeygolu420...,
Refer below sample.
Database
I have made use of the following table Customers with the schema as follows. CustomerId is an Auto-Increment (Identity) column.
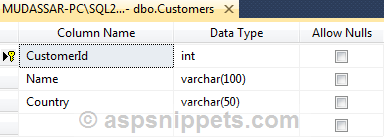
I have already inserted few records in the table.
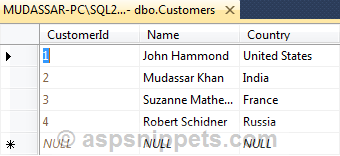
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult AjaxMethod(string name)
{
List<SelectListItem> customers = (from customer in this.Context.Customers.Take(10)
select new SelectListItem
{
Value = (customer.CustomerId).ToString(),
Text = customer.Name
}).ToList();
return Json(customers);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<style type="text/css">
body {
font-family: Arial;
font-size: 10pt;
}
</style>
</head>
<body>
<form method="post" asp-controller="Home" asp-action="Index">
<select id="ddlCustomers" multiple="multiple">
<option value="0">--Select Customer--</option>
</select>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/css/select2.min.css" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/js/select2.min.js">
</script>
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
url: "/Home/AjaxMethod",
data: '{}',
success: function (response) {
var ddlCustomers = $("#ddlCustomers");
ddlCustomers.empty();
$.each(response, function () {
ddlCustomers.append($("<option></option>").val(this['Value']).html(this['Text']));
});
$("#ddlCustomers").select2();
},
failure: function (response) {
alert(response.d);
},
error: function (response) {
alert(response.d);
}
});
});
</script>
</form>
</body>
</html>
For more details on multiselect dropdownlist refer