Hi syedtalib4,
Check this example. Now please take its reference and correct your code.
DataBase
I have made use of the following table Customers with the schema as follows.
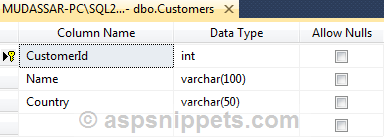
I have already inserted few records in the table.
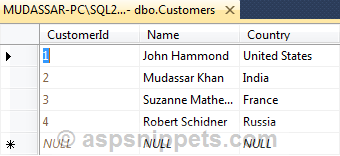
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div>
<asp:HiddenField ID="chkChecked" runat="server" />
<asp:Button runat="server" ID="button4" OnClick="button4_Click" Text="Delete" />
<br />
<br />
<table id="tblEmployee">
<thead>
<tr>
<th>
<input type="checkbox" class="checkAll" />
</th>
<th>
ID
</th>
<th>
Name
</th>
<th>
Country
</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(document).on('click', '.checkAll', function (e) {
var table = $(this).closest('table');
table.find(':checkbox').not(this).prop('checked', this.checked);
});
$(function () {
GetCustomers();
});
function GetCustomers() {
$.ajax({
type: "POST",
url: "CS.aspx/GetCustomers",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (data) {
var employeetable = $('#tblEmployee tbody');
$(data.d).each(function (index, emp) {
employeetable.append('<tr>' +
'<td><input type="checkbox" name="chkdelete" id="chkdelete" class="employeeIdsToDelete" value=' + emp.CustomerId + '></td>' +
'<td>' + emp.CustomerId + '</td>' +
'<td>' + emp.Name + '</td>' +
'<td>' + emp.Country + '</td>' +
'</tr>');
});
},
failure: function (response) { alert(response.d); },
error: function (response) { alert(response.responseText); }
});
}
</script>
Namespaces
C#
using System.Web.Services;
using System.Data.SqlClient;
using System.Configuration;
using System.Data;
VB.Net
Imports System.Web.Services
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Data
Code
C#
[WebMethod]
public static List<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
string query = "SELECT CustomerId, Name, Country FROM Customers";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
customers.Add(new Customer
{
CustomerId = Convert.ToInt32(sdr["CustomerId"]),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
}
}
con.Close();
return customers;
}
}
protected void button4_Click(object sender, EventArgs e)
{
string[] checkedIds = Request.Form["chkdelete"].Split(',');
foreach (string id in checkedIds)
{
DeletebyEmpId(Convert.ToInt32(id));
}
}
public static void DeletebyEmpId(int ID)
{
string connectionstring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection connection = new SqlConnection(connectionstring))
{
SqlCommand cmd = new SqlCommand("Delete from Customers Where CustomerId=@ID", connection);
SqlParameter param = new SqlParameter("@ID", ID);
cmd.Parameters.Add(param);
connection.Open();
cmd.ExecuteNonQuery();
connection.Close();
}
}
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
VB.Net
<WebMethod>
Public Shared Function GetCustomers() As List(Of Customer)
Dim customers As List(Of Customer) = New List(Of Customer)()
Dim query As String = "SELECT CustomerId, Name, Country FROM CustomerTest"
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand(query)
cmd.Connection = con
con.Open()
Using sdr As SqlDataReader = cmd.ExecuteReader()
While sdr.Read()
customers.Add(New Customer With {.CustomerId = Convert.ToInt32(sdr("CustomerId")), .Name = sdr("Name").ToString(), .Country = sdr("Country").ToString()})
End While
End Using
End Using
con.Close()
Return customers
End Using
End Function
Protected Sub button4_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim checkedIds As String() = Request.Form("chkdelete").Split(","c)
For Each id As String In checkedIds
DeletebyEmpId(Convert.ToInt32(id))
Next
End Sub
Public Shared Sub DeletebyEmpId(ByVal ID As Integer)
Dim connectionstring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using connection As SqlConnection = New SqlConnection(connectionstring)
Dim cmd As SqlCommand = New SqlCommand("Delete from CustomerTest Where CustomerId=@ID", connection)
Dim param As SqlParameter = New SqlParameter("@ID", ID)
cmd.Parameters.Add(param)
connection.Open()
cmd.ExecuteNonQuery()
connection.Close()
End Using
End Sub
Public Class Customer
Public Property CustomerId As Integer
Public Property Name As String
Public Property Country As String
End Class
Screenshot
