Hi akshay806,
Check this example. Now please take its reference and correct your code.
To consume ASMX Web Service refer the below article.
Database
For this sample I have created a simple table tblFiles with the following structure.
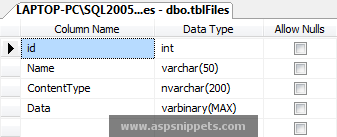
You can download the database table SQL by clicking the download link below.
Download SQL file
ASMX Webservice
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Services;
/// <summary>
/// Summary description for FileUploadService
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class FileUploadService : System.Web.Services.WebService
{
public FileUploadService()
{
//Uncomment the following line if using designed components
//InitializeComponent();
}
[WebMethod]
public void UploadFile(string name, string contentType, byte[] byteArray)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO tblFiles VALUES(@Name,@ContentType,@Data)", con))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@ContentType", contentType);
cmd.Parameters.AddWithValue("@Data", byteArray);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
}
VB.Net
Imports System.Web
Imports System.Web.Services
Imports System.Web.Services.Protocols
Imports System.Data
Imports System.Data.SqlClient
' To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
' <System.Web.Script.Services.ScriptService()> _
<WebService(Namespace:="http://tempuri.org/")> _
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)> _
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Public Class FileUploadService
Inherits System.Web.Services.WebService
<WebMethod()>
Public Sub UploadFile(ByVal name As String, ByVal contentType As String, ByVal byteArray As Byte())
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("INSERT INTO tblFiles VALUES(@Name,@ContentType,@Data)", con)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@ContentType", contentType)
cmd.Parameters.AddWithValue("@Data", byteArray)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
End Sub
End Class
HTML
<asp:FileUpload ID="fuUpload" runat="server" />
<asp:Button ID="btnSave" Text="Save" runat="server" OnClick="Save" />
Namespace
You will need to import the following namespace.
C#
using System.IO;
VB.Net
Imports System.IO
Code
C#
protected void Save(object sender, EventArgs e)
{
if (fuUpload.HasFile)
{
string name = fuUpload.FileName;
string contentType = fuUpload.PostedFile.ContentType;
byte[] byteArray;
using (Stream stream = fuUpload.PostedFile.InputStream)
{
using (BinaryReader binaryReader = new BinaryReader(stream))
{
byteArray = binaryReader.ReadBytes((Int32)stream.Length);
}
}
UploadService.FileUploadServiceSoapClient soapClient = new UploadService.FileUploadServiceSoapClient();
soapClient.UploadFile(name, contentType, byteArray);
}
}
VB.Net
Protected Sub Save(ByVal sender As Object, ByVal e As EventArgs)
If fuUpload.HasFile Then
Dim name As String = fuUpload.FileName
Dim contentType As String = fuUpload.PostedFile.ContentType
Dim byteArray As Byte()
Using stream As Stream = fuUpload.PostedFile.InputStream
Using binaryReader As BinaryReader = New BinaryReader(stream)
byteArray = binaryReader.ReadBytes(CType(stream.Length, Int32))
End Using
End Using
Dim soapClient As UploadService.FileUploadServiceSoapClient = New UploadService.FileUploadServiceSoapClient()
soapClient.UploadFile(name, contentType, byteArray)
End If
End Sub
After saving the database table looks like below.
Screenshot
