Hi azfar,
Refer below sample.
Database
For this example i have used table named tblFiles whose schema is defined as follows.
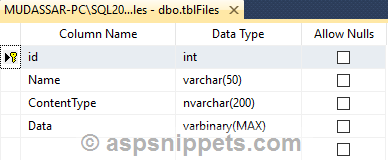
You can download the database table SQL by clicking the download link below.
Download SQL file
For more details refer below article.
HTML
<asp:Button Text="Save" runat="server" OnClick="Save" />
Namespaces
C#
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
using System.IO;
VB.Net
Imports System.Data
Imports System.IO
Imports System.Data.SqlClient
Code
C#
protected void Save(object sender, EventArgs e)
{
DataTable dt = GetData("SELECT * FROM tblFiles WHERE ContentType = 'image/jpeg'");
for (int i = 0; i < dt.Rows.Count; i++)
{
byte[] bytes = (byte[])dt.Rows[i]["Data"];
File.WriteAllBytes(Server.MapPath("~/Files/" + dt.Rows[i]["Id"] + ".jpg"), bytes);
}
}
private DataTable GetData(string query)
{
DataTable dt = new DataTable();
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
sda.SelectCommand = cmd;
sda.Fill(dt);
}
}
return dt;
}
}
VB.Net
Protected Sub Save(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
Dim dt As DataTable = GetData("SELECT * FROM tblFiles WHERE ContentType = 'image/jpeg'")
For i As Integer = 0 To dt.Rows.Count - 1
Dim bytes As Byte() = CType(dt.Rows(i)("Data"), Byte())
File.WriteAllBytes(Server.MapPath("~/Files/" & dt.Rows(i)("Id") & ".jpg"), bytes)
Next
End Sub
Private Function GetData(ByVal query As String) As DataTable
Dim dt As DataTable = New DataTable()
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using cmd As SqlCommand = New SqlCommand(query)
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.CommandType = CommandType.Text
cmd.Connection = con
sda.SelectCommand = cmd
sda.Fill(dt)
End Using
End Using
Return dt
End Using
End Function