You will need to use a Generic Handler ASHX file and not ASPX file.
Database
I have made use of the following table Customers with the schema as follows.
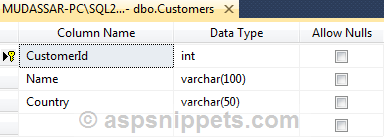
I have already inserted few records in the table.
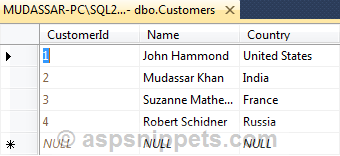
You can download the database table SQL by clicking the download link below.
Generic Handler
<%@ WebHandler Language="C#" Class="JSONHandlerCS" %>
using System;
using System.Web;
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
using System.Collections.Generic;
using System.Web.Script.Serialization;
public class JSONHandlerCS : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
string callback = context.Request["callback"];
int customerId = 0;
int.TryParse(context.Request["customerId"], out customerId);
string json = this.GetCustomersJSON(customerId);
if (!string.IsNullOrEmpty(callback))
{
json = string.Format("{0}({1});", callback, json);
}
context.Response.ContentType = "text/json";
context.Response.Write(json);
}
private string GetCustomersJSON(int customerId)
{
List<object> customers = new List<object>();
using (SqlConnection conn = new SqlConnection())
{
conn.ConnectionString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandText = "SELECT * FROM Customers WHERE CustomerId = @CustomerId OR @CustomerId = 0";
cmd.Parameters.AddWithValue("@CustomerId", customerId);
cmd.Connection = conn;
conn.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
customers.Add(new
{
CustomerId = sdr["CustomerId"],
Name = sdr["Name"],
Country = sdr["Country"]
});
}
}
conn.Close();
}
return (new JavaScriptSerializer().Serialize(customers));
}
}
public bool IsReusable
{
get
{
return false;
}
}
}
Screenshot
