Here I have used ASP.Net Ajax Timer control to Save the Image after each 5 minutes.
Please refer this code.
Ref:
Capture Screenshot (Snapshot) Image of Website (Web Page) in ASP.Net using C# and VB.Net
HTML
<asp:ScriptManager runat="server" />
<asp:Timer ID="Timer1" runat="server" OnTick="OnTick" Interval="10000" Enabled="false">
</asp:Timer>
<asp:TextBox ID="txtUrl" runat="server" Text="" />
<asp:Button ID="btnCapture" Text="Capture" runat="server" OnClick="Capture" />
<br />
<asp:Image ID="imgScreenShot" runat="server" Height="500" Width="700" Visible="false" />
Namespaces
using System.IO;
using System.Drawing;
using System.Threading;
using System.Windows.Forms;
using System.Configuration;
using System.Data.SqlClient;
using System.Data;
C#
protected void OnTick(object sender, EventArgs e)
{
string url = txtUrl.Text.Trim();
if (!string.IsNullOrEmpty(url))
{
Thread thread = new Thread(delegate()
{
using (WebBrowser browser = new WebBrowser())
{
browser.ScrollBarsEnabled = false;
browser.AllowNavigation = true;
browser.Navigate(url);
browser.Width = 1024;
browser.Height = 768;
browser.DocumentCompleted += new WebBrowserDocumentCompletedEventHandler(DocumentCompleted);
while (browser.ReadyState != WebBrowserReadyState.Complete)
{
System.Windows.Forms.Application.DoEvents();
}
}
});
thread.SetApartmentState(ApartmentState.STA);
thread.Start();
thread.Join();
}
}
public void Capture(object sender, EventArgs e)
{
this.Timer1.Enabled = true;
}
private void DocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e)
{
WebBrowser browser = sender as WebBrowser;
byte[] bytes;
using (Bitmap bitmap = new Bitmap(browser.Width, browser.Height))
{
browser.DrawToBitmap(bitmap, new Rectangle(0, 0, browser.Width, browser.Height));
using (MemoryStream stream = new MemoryStream())
{
bitmap.Save(stream, System.Drawing.Imaging.ImageFormat.Png);
bytes = stream.ToArray();
imgScreenShot.Visible = true;
imgScreenShot.ImageUrl = "data:image/png;base64," + Convert.ToBase64String(bytes);
}
}
string query = "INSERT INTO tblFiles(Name, ContentType, Data) VALUES (@Name, @ContentType, @Data)";
string constr = ConfigurationManager.ConnectionStrings["conString"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Parameters.Add("@Name", SqlDbType.VarChar).Value = txtUrl.Text.Split(':')[1].Replace("/", "");
cmd.Parameters.Add("@ContentType", SqlDbType.VarChar).Value = "image/png";
cmd.Parameters.Add("@Data", SqlDbType.Binary).Value = bytes;
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
For this article I have created a simple table with the following structure.
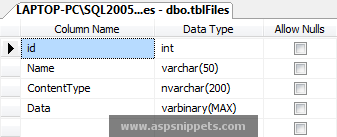
You can download the database table SQL by clicking the download link below.
Download SQL file