Hi RPA,
Please refer below sample.
Database
For this Sample I have created a new database named LoginDB which contains the following table named Users in it.
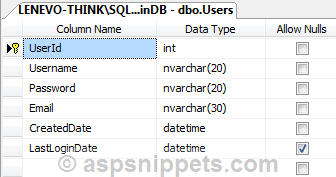
I have already inserted few records in the table.

HTML
<table border="0" cellpadding="2" cellspacing="0">
<tr>
<td>UserName:</td>
<td><asp:TextBox ID="txtUserName" runat="server" /></td>
</tr>
<tr>
<td>Password:</td>
<td><asp:TextBox ID="txtPassword" runat="server" TextMode="Password" /></td>
</tr>
<tr>
<td></td>
<td><asp:Button ID="btnSubmit" Text="Submit" runat="server" OnClick="Submit" /></td>
</tr>
<tr>
<td>Output:</td>
<td><asp:Label ID="lblOutput" runat="server" /></td>
</tr>
</table>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.IO;
using System.Net;
using System.Text;
using System.Web.Script.Serialization;
using System.ServiceModel;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.IO
Imports System.Net
Imports System.Text
Imports System.Web.Script.Serialization
Imports System.ServiceModel
Code
C#
Default
protected void Submit(object sender, EventArgs e)
{
string serviceUrl = "http://localhost:17754/Services/Service.svc";
object input = new
{
userName = txtUserName.Text.Trim(),
password = txtPassword.Text.Trim()
};
string inputJson = (new JavaScriptSerializer()).Serialize(input);
HttpWebRequest httpRequest = (HttpWebRequest)WebRequest.Create(new Uri(serviceUrl + "/ValidateLogin"));
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
httpRequest.Method = "POST";
byte[] bytes = Encoding.UTF8.GetBytes(inputJson);
using (Stream stream = httpRequest.GetRequestStream())
{
stream.Write(bytes, 0, bytes.Length);
stream.Close();
}
using (HttpWebResponse httpResponse = (HttpWebResponse)httpRequest.GetResponse())
{
using (Stream stream = httpResponse.GetResponseStream())
{
string json = (new StreamReader(stream)).ReadToEnd();
lblOutput.Text = (new JavaScriptSerializer()).Deserialize<Dictionary<string, string>>(json).Values.ToArray()[0];
}
}
}
Service
public class Service : IService
{
public string ValidateLogin(string userName, string password)
{
bool isValid = false;
// Validation code.
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT COUNT(*) FROM Users WHERE UserName = @UserName and Password = @Password", con))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@UserName", userName);
cmd.Parameters.AddWithValue("@Password", password);
con.Open();
isValid = Convert.ToInt32(cmd.ExecuteScalar()) == 0 ? false : true;
con.Close();
}
}
return isValid.ToString();
}
}
IService
[ServiceContract]
public interface IService
{
[OperationContract]
string ValidateLogin(string userName, string password);
}
VB.Net
Default
Protected Sub Submit(ByVal sender As Object, ByVal e As EventArgs)
Dim serviceUrl As String = "http://localhost:17754/Services/Service.svc"
Dim input As Object = New With {
Key .userName = txtUserName.Text.Trim(),
Key .password = txtPassword.Text.Trim()
}
Dim inputJson As String = (New JavaScriptSerializer()).Serialize(input)
Dim httpRequest As HttpWebRequest = CType(WebRequest.Create(New Uri(serviceUrl & "/ValidateLogin")), HttpWebRequest)
httpRequest.Accept = "application/json"
httpRequest.ContentType = "application/json"
httpRequest.Method = "POST"
Dim bytes As Byte() = Encoding.UTF8.GetBytes(inputJson)
Using stream As Stream = httpRequest.GetRequestStream()
stream.Write(bytes, 0, bytes.Length)
stream.Close()
End Using
Using httpResponse As HttpWebResponse = CType(httpRequest.GetResponse(), HttpWebResponse)
Using stream As Stream = httpResponse.GetResponseStream()
Dim json As String = (New StreamReader(stream)).ReadToEnd()
lblOutput.Text = (New JavaScriptSerializer()).Deserialize(Of Dictionary(Of String, String))(json).Values.ToArray()(0)
End Using
End Using
End Sub
Service
Public Class Service
Implements IService
Public Function ValidateLogin(ByVal userName As String, ByVal password As String) As String Implements IService.ValidateLogin
Dim isValid As Boolean = False
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT COUNT(*) FROM Users WHERE UserName = @UserName and Password = @Password", con)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@UserName", userName)
cmd.Parameters.AddWithValue("@Password", password)
con.Open()
isValid = If(Convert.ToInt32(cmd.ExecuteScalar()) = 0, False, True)
con.Close()
End Using
End Using
Return isValid.ToString()
End Function
End Class
IService
<ServiceContract()>
Interface IService
<OperationContract>
Function ValidateLogin(ByVal userName As String, ByVal password As String) As String
End Interface
Screenshot

Note: I have created this sample with reference from Perform HTTP POST to REST WCF Service (SVC) in ASP.Net using C# and VB.Net